To set up alerts for when the Linx VM memory exceeds a certain limit, you can use the following script and you can change the limit value and the folders to whatever is convenient for you:
#!/bin/bash
# set alert level 90% is default
ALERT=90
# Exclude list of unwanted monitoring, if several partions then use "|" to separate the partitions.
EXCLUDE_LIST="/auto/ripper|loop"
LIST=( "example1@gmail.com" "example1@gmail.com" "example1@gmail.com" )
sender="example1@gmail.com"
gmpwd="smtp password goes here"
sub="Virtual Machine ran out of space"
#
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
#
main_prog() {
while read -r output;
do
#Get public ip of ur VM
publicip=`dig TXT +short o-o.myaddr.l.google.com @ns1.google.com`
usep=$(echo "$output" | awk '{ print $1}' | cut -d'%' -f1)
partition=$(echo "$output" | awk '{print $2}')
if [ $usep -ge $ALERT ] ; then
echo "Running out of space \"$partition ($usep%)\" on server $(hostname), $(date)\nPublic IP: $publicip" > "alert.txt"
body="Running out of space \"$partition ($usep%)\" on server $(hostname), $(date)\nPublic IP: $publicip"
for i in "${LIST[@]}"
do
receiver=$i
file=alert.txt
MIMEType=`file --mime-type "$file" | sed 's/.*: //'`
curl -s --url 'smtps://smtp.gmail.com:465' --ssl-reqd \
--mail-from $sender \
--mail-rcpt $receiver\
--user $sender:$gmpwd \
-F '=(;type=multipart/mixed' -F "=$body;type=text/plain" -F "file=@$file;type=$MIMEType;encoder=base64" -F '=)' \
-H "Subject: $sub" -H "From: Alerts <$sender>" -H "To: $i"
done
fi
done
}
if [ "$EXCLUDE_LIST" != "" ] ; then
df -H | grep -vE "^Filesystem|tmpfs|cdrom|${EXCLUDE_LIST}" | awk '{print $5 " " $6}' | main_prog
else
df -H | grep -vE "^Filesystem|tmpfs|cdrom" | awk '{print $5 " " $6}' | main_prog
fi
This is a Bash script that checks the disk space usage on a Linux machine and sends an email alert if the usage exceeds a certain threshold. Here is a breakdown of the different sections of the script:
- The first line (
#!/bin/bash
) specifies the interpreter that should be used to run the script. - The
ALERT
variable sets the threshold for disk usage at which an alert should be triggered. The default value is 90%. - The
EXCLUDE_LIST
variable contains a list of partitions that should be excluded from monitoring. In this example,/auto/ripper
andloop
are excluded. - The
LIST
array contains a list of email addresses that should receive the alert. The script sends the alert to each address in the array. - The
sender
variable contains the email address of the sender. - The
gmpwd
variable contains the password for the SMTP server used to send the email. - The
sub
variable contains the subject of the email alert. - The
main_prog
function is the main body of the script. It reads the output of thedf
command (which displays information about file system disk space usage) and checks if the usage percentage for each partition exceeds the alert threshold. If the usage is above the threshold, it sends an email alert to each address in theLIST
array. - The
if
statement checks if theEXCLUDE_LIST
variable is set, and excludes the specified partitions from monitoring if it is. - The
df
command is piped togrep
, which filters out any unwanted partitions specified in theEXCLUDE_LIST
variable. The resulting output is then piped toawk
, which extracts the usage percentage and partition name for each file system. - The output of
awk
is passed to themain_prog
function for further processing.
An example of Alert received (threshold was 80%):
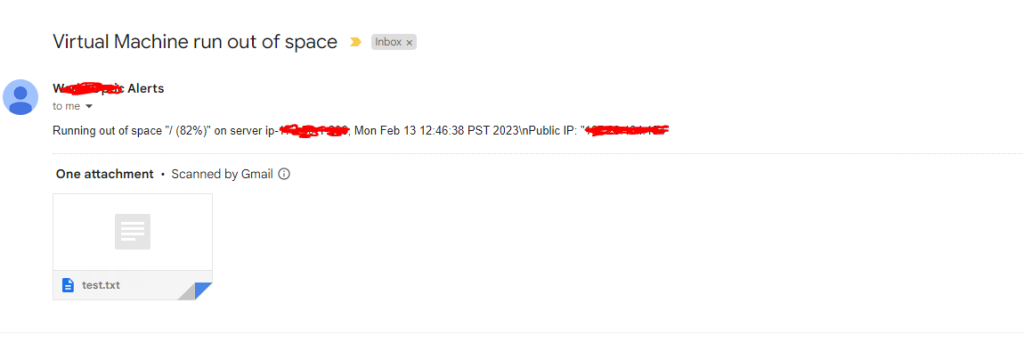
Overall, this script provides a simple way to monitor disk space usage on a Linux machine and alert administrators when usage exceeds a certain threshold.